angular-响应式表单验证
Created At :
Views 👀 :
需求:新建品名为冲压小件的排产单后,右键引排产单明细时,查询的字段的显示:
如果品名是冲压小件的,添加弹窗只显示编号,长度,展宽,厚度及块数(且编号与厚度不必填)
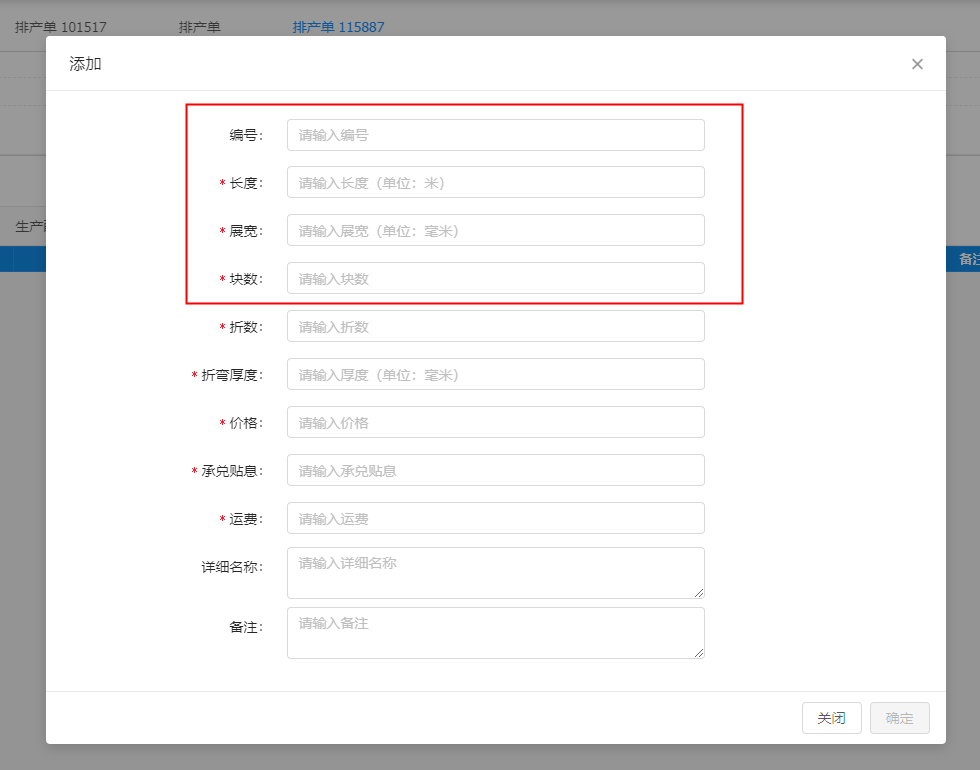
解决方案:
使用clearValidators():清空同步验证器列表及updateValueAndValidity():重新计算控件的值和验证状态。
注:在运行时添加或删除验证器时,必须调用 updateValueAndValidity() 以使新验证生效。
使用使用clearValidators()和updateValueAndValidity()使得折数,折弯厚度,价格等必填字段在满足品名为冲压小件的排产单引排产明细时,实现不必填的状态。
1 2 3 4 5 6 7 8 9 10 11 12
| if(this.paichanDataName==='冲压小件'){ this.formGroup.get('zheshu').clearValidators(); this.formGroup.get('zheshu').updateValueAndValidity(); this.formGroup.get('zhewanhd').clearValidators(); this.formGroup.get('zhewanhd').updateValueAndValidity(); this.formGroup.get('price').clearValidators(); this.formGroup.get('price').updateValueAndValidity(); this.formGroup.get('tiexi').clearValidators(); this.formGroup.get('tiexi').updateValueAndValidity(); this.formGroup.get('yunfei').clearValidators(); this.formGroup.get('yunfei').updateValueAndValidity(); }
|
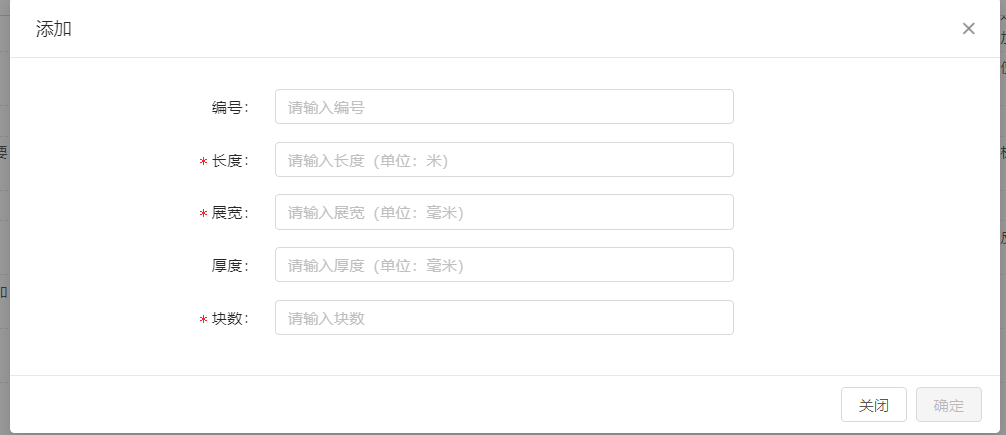
学习:
验证器静态方法如下:
Validators.min(n):该验证器要求控件值最小值为n(n为数字)。
Validators.max(n):该验证器要求控件值最大值为n(n为数字)。
Validators.required:该验证器要求控件具有非空值,即该控件所表示的字段必填。
Validators.minLength(n):该验证器要求控件值的最小长度为n(n为数字)。
Validators.maxLength(n):该验证器要求控件值的最大长度为n(n为数字)。
validators:用于同步确定此控件的有效性的函数或函数数组。
clearValidators():清空同步验证器列表。
clearValidators(): void。
参数:没有参数。
返回值:void。
注:
在运行时添加或删除验证器时,必须调用 updateValueAndValidity() 以使新验证生效。
updateValueAndValidity():重新计算控件的值和验证状态。
updateValueAndValidity(opts: { onlySelf?: boolean; emitEvent?: boolean; } = {}): void
参数:
opts object 配置选项确定控件在应用更新和有效性检查后如何传播更改和发出事件。
①onlySelf :当 true 时,仅更新此控件。当 false 或未提供时,更新所有直接祖先。默认为 false。
②emitEvent :当为 true 或不提供(默认)时,statusChanges 和 valueChanges 可观察对象会在控件更新时发出具有最新状态和值的事件。当 false 时,不会发出事件。
可选值。默认值为 {}。
返回值:void
默认情况下,它还会更新其祖先的值和有效性。
什么是响应式表单?
响应式表单提供了一种模型驱动的方式来处理表单输入,其中的值会随时间而变化。
响应式表单使用显示的,不可变的方式,管理表单在特定时间点上的状态。对表单状态的每一次变更都会返回一个新的状态,这样可以在变化时维护模型的整体性。
响应式表单的使用:
单个表单控件:
1 2 3 4 5 6 7 8 9
| import { ReactiveFormsModule } from '@angular/forms';
@NgModule({ imports: [ ReactiveFormsModule ], }) export class AppModule { }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| import { Component, OnInit } from '@angular/core'; import { FormControl } from '@angular/forms';
@Component({ selector: 'app-welcome', templateUrl: './welcome.component.html', styleUrls: ['./welcome.component.css'] }) export class WelcomeComponent implements OnInit {
name = new FormControl('');
constructor() { }
ngOnInit() { }
}
|
步骤3: 在模板中注入该控件.
1 2 3 4 5 6
| <label> FormControl测试:<input nz-input [formControl]="name" placeholder="输入测试信息"> </label> <label> 输入的测试信息为:{{name.value}} </label>
|
表单控件分组
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| import { Component } from '@angular/core'; import { FormGroup, FormControl } from '@angular/forms';
@Component({ selector: 'app-profile-editor', templateUrl: './profile-editor.component.html', styleUrls: ['./profile-editor.component.css'] }) export class ProfileEditorComponent { profileForm = new FormGroup({ firstName: new FormControl(''), lastName: new FormControl(''), }); }
|
这个表单组跟踪其中每个控件的状态和值变化,所以如果其中的某个控件的状态或值变化了,父控件会发出一次新的状态变更或值变更事件。
该控件组的模型来自于它的所有成员。在定义了这个模型之后,必须更新模板,来把该模型反映到视图中。
1 2 3 4 5 6 7 8 9 10 11 12 13
| <form [formGroup]="profileForm"> <label> First Name: <input type="text" formControlName="firstName"> </label>
<label> Last Name: <input type="text" formControlName="lastName"> </label>
</form>
|
注:
模型中创建的FormGroup实例通过FromGroup指令绑定到form元素上,在该模型和表单中的输入框之间创建一个通讯层。
由FormControlName指令提供的formControlName属性把每个输入框和FormGroup中定义的表单控件绑定起来。这些表单控件会和相应的元素通讯,它们还把更改传递给FormGroup,这个FormGroup是模型值的权威数据源。
1
| import { FormBuilder } from '@angular/forms';
|
1 2
| constructor(private fb: FormBuilder) { }
|
步骤3: 生成表单控件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| import { Component } from '@angular/core'; import { FormBuilder } from '@angular/forms';
@Component({ selector: 'app-profile-editor', templateUrl: './profile-editor.component.html', styleUrls: ['./profile-editor.component.css'] }) export class ProfileEditorComponent { profileForm = this.fb.group({ firstName: [''], lastName: [''], address: this.fb.group({ street: [''], city: [''], state: [''], zip: [''] }), });
constructor(private fb: FormBuilder) { } }
|
表单验证:
响应式表单包含了一组开箱即用的常用验证器函数。这些函数接收一个控件,用以验证并根据验证结果返回一个错误对象或空值。
步骤1: 创建表单控件
步骤2: 导入 验证器函数
1 2
| import { Validators } from '@angular/forms';
|
步骤3: 添加验证器静态方法
将验证器静态方法设置为表单控件值数组的第二项。
验证器静态方法如下:
Validators.min(n):该验证器要求控件值最小值为n(n为数字)。
Validators.max(n):该验证器要求控件值最大值为n(n为数字)。
Validators.required:该验证器要求控件具有非空值,即该控件所表示的字段必填。
Validators.minLength(n):该验证器要求控件值的最小长度为n(n为数字)。
Validators.maxLength(n):该验证器要求控件值的最大长度为n(n为数字)。